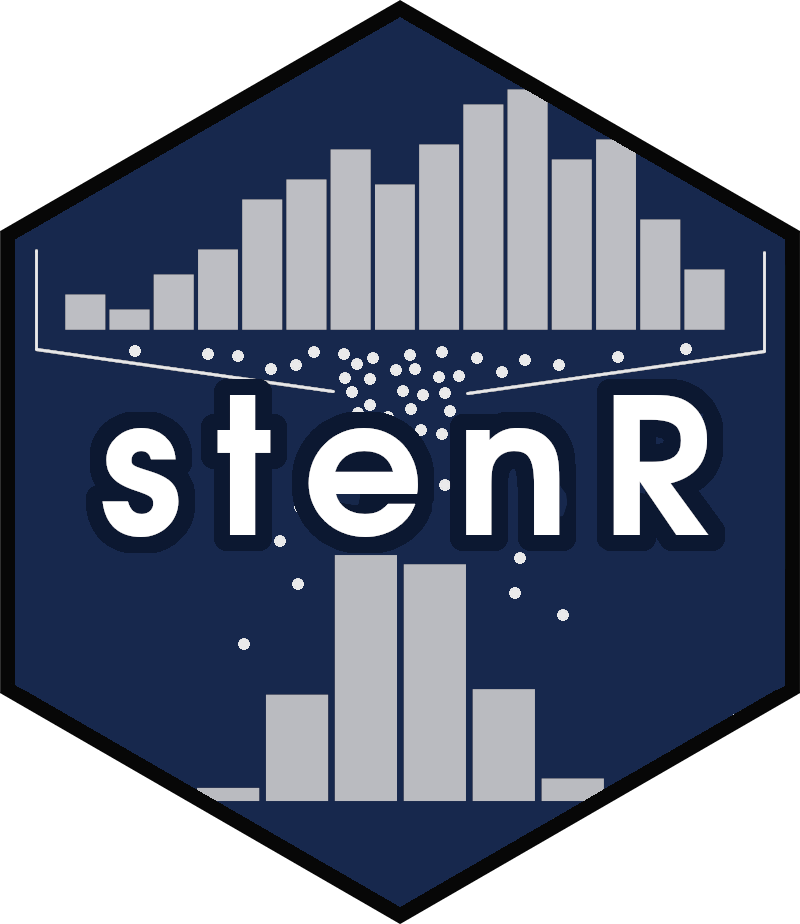
Normalize scores using GroupedFrequencyTables or GroupedScoreTables
normalize_scores_grouped.Rd
Normalize scores using either GroupedFrequencyTable
or
GroupedScoreTable
for one or more variables. Given data.frame should also
contain columns used in GroupingConditions
attached to the table
Usage
normalize_scores_grouped(
data,
vars,
...,
what,
retain = FALSE,
group_col = NULL,
.dots = list()
)
Arguments
- data
data.frame object containing raw scores
- vars
names of columns to normalize. Length of vars need to be the same as number of tables provided to either
...
or.dots
- ...
GroupedFrequencyTable
orGroupedScoreTable
objects to be used for normalization. They should be provided in the same order asvars
- what
the values to get. One of either:
quan
- the quantile of x in the raw score distributionZ
- normalized Z score for the x raw scorename of the scale calculated in
GroupedScoreTables
provided to...
or.dots
argument
- retain
either boolean:
TRUE
if all columns in thedata
are to be retained,FALSE
if none; or character vector with names of columns to be retained- group_col
name of the column for name of the group each observation was qualified into. If left as default
NULL
, they won't be returned.- .dots
GroupedFrequencyTable
orGroupedScoreTable
objects provided as a list, instead of individually in...
.
See also
Other score-normalization functions:
normalize_scores_df()
,
normalize_scores_scoring()
,
normalize_score()
Examples
# setup - create necessary objects #
suppressMessages({
age_grouping <- GroupConditions(
conditions_category = "Age",
"below 22" ~ age < 22,
"23-60" ~ age >= 23 & age <= 60,
"above 60" ~ age > 60
)
sex_grouping <- GroupConditions(
conditions_category = "Sex",
"Male" ~ sex == "M",
"Female" ~ sex == "F"
)
NEU_gft <- GroupedFrequencyTable(
data = IPIP_NEO_300,
conditions = list(age_grouping, sex_grouping),
var = "N"
)
NEU_gst <- GroupedScoreTable(
NEU_gft,
scale = list(STEN, STANINE)
)
})
#### normalize scores ####
# to Z score or quantile using GroupedFrequencyTable
normalized_to_quan <- normalize_scores_grouped(
IPIP_NEO_300,
vars = "N",
NEU_gft,
what = "quan",
retain = c("sex", "age")
)
# only 'sex' and 'age' are retained
head(normalized_to_quan)
#> sex age N
#> 1: F 25 62.53234
#> 2: F 18 77.32835
#> 3: F 16 77.32835
#> 4: M 23 58.89060
#> 5: F 25 38.13066
#> 6: M 26 80.09245
# to StandardScale attached to GroupedScoreTable
normalized_to_STEN <- normalize_scores_grouped(
IPIP_NEO_300,
vars = "N",
NEU_gst,
what = "stanine",
retain = FALSE,
group_col = "sex_age_group"
)
# none is retained, 'sex_age_group' is created
head(normalized_to_STEN)
#> sex_age_group N
#> 1: 23-60:Female 6
#> 2: below 22:Female 6
#> 3: below 22:Female 6
#> 4: 23-60:Male 5
#> 5: 23-60:Female 4
#> 6: 23-60:Male 7