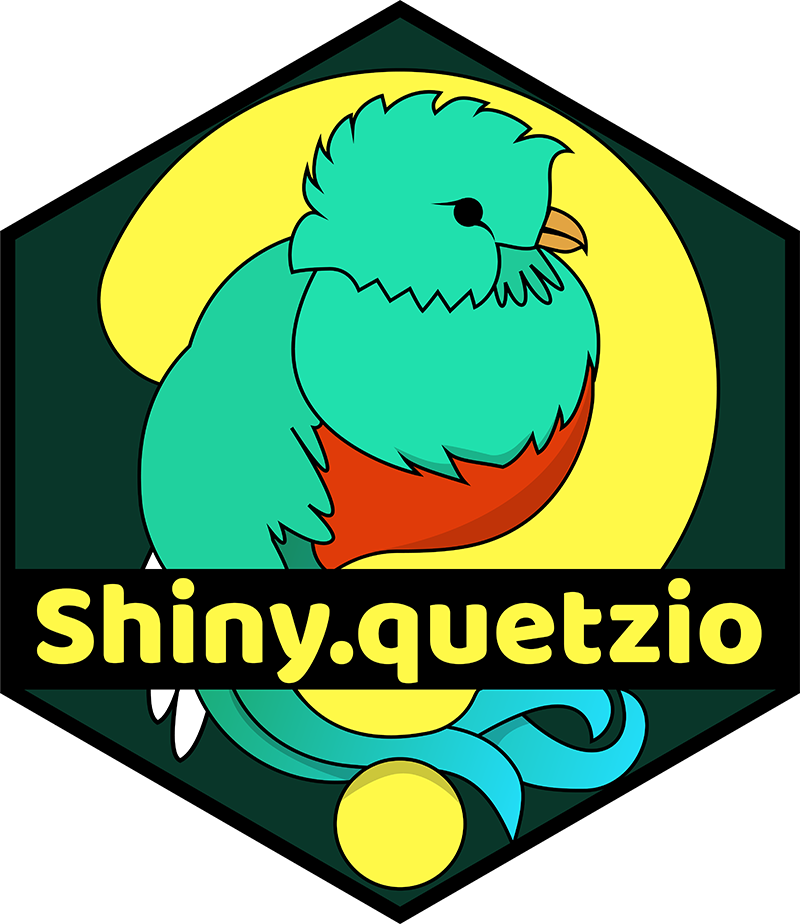
Update Quetzio labels triggered by reactive value change
Quetzio_label_update.Rd
Update Quetzio labels triggered by reactive value change
Usage
Quetzio_label_update(
Quetzio,
trigger,
source_method,
source_yaml = NULL,
source_gsheet_id = NULL,
source_gsheet_sheetname = NULL,
source_object = NULL,
name = NULL
)
# S3 method for Quetzio
Quetzio_label_update(
Quetzio,
trigger,
source_method,
source_yaml = NULL,
source_gsheet_id = NULL,
source_gsheet_sheetname = NULL,
source_object = NULL
)
# S3 method for QuetzioLink
Quetzio_label_update(
Quetzio,
trigger,
source_method,
source_yaml = NULL,
source_gsheet_id = NULL,
source_gsheet_sheetname = NULL,
source_object = NULL,
name = NULL
)
Arguments
- Quetzio
object of class
Quetzio
orQuetzio_link
- trigger
reactive which will trigger the update. It needs to take values linked to the changes in the source
- source_method
character string specifying in what form the source config file will be provided. Can be either 'gsheet', 'yaml' or 'raw'. Necessity of other arguments is dependent on this choice.
- source_yaml
path to the source yaml file
- source_gsheet_id
id of the source googlesheet file
- source_gsheet_sheetname
name of the source spreadsheet
- source_object
object of class
list
(similiar in structure to 'yaml' source) ordata.frame
(similiar in structure to 'googlesheet' source) to be the source of questions. You can create a sample data.frame withcreate_survey_source()
. Needed whensource_method == 'raw'
- name
string indicating in which questionnaire the questions to update are located. Used with
QuetzioLink
objects
Examples
## only run examples in interactive environment
if (interactive()) {
library(shiny)
library(shiny.quetzio)
ui <- fluidPage(
# some input to trigger label update
selectizeInput("gender", "What is your gender?",
choices = c("Male" = "M",
"Female" = "F",
"I identify as neither of above" = "O",
"Prefer not to say" = "NI"),
selected = "NI"),
tags$hr(),
# quetzio to update labels
Quetzio_UI("updating_labels")
)
server <- function(input, output, session) {
quetzio <- Quetzio_create(
source_method = "raw",
source_object = quetzio_examples$questions_lists$gender_update,
module_id = "updating_labels"
)
# trigger need to be reactive
gender_react <- reactive(input$gender)
# update labels method call
Quetzio_label_update(
Quetzio = quetzio,
trigger = gender_react,
source_method = "raw",
source_object = quetzio_examples$label_update$gender_update
)
}
shinyApp(ui, server)
}
## only run examples in interactive environment
if (interactive()) {
library(shiny)
library(shiny.quetzio)
ui <- fluidPage(
# some input to trigger label update
selectizeInput("gender", "What is your gender?",
choices = c("Male" = "M",
"Female" = "F",
"I identify as neither of above" = "O",
"Prefer not to say" = "NI"),
selected = "NI"),
tags$hr(),
# quetzio to update labels
QuetzioLink_UI("labels_link")
)
server <- function(input, output, session) {
quetzio_link <- QuetzioLink_create(
quetzio_2nd = Quetzio_create(
source_method = "raw",
source_object = quetzio_examples$questions_lists$simple_quetzio,
module_id = "second_in_link"
),
gender = Quetzio_create(
source_method = "raw",
source_object = quetzio_examples$questions_lists$gender_update,
module_id = "updating_labels"
),
link_id = "labels_link")
# trigger need to be reactive
gender_react <- reactive(input$gender)
# update labels method call
Quetzio_label_update(
Quetzio = quetzio_link,
# you need to provide the name of the quetzio_server in link
# where you need to update labels
name = "gender",
# the trigger needs to be reactive, but without the parentheses
trigger = gender_react,
source_method = "raw",
source_object = quetzio_examples$label_update$gender_update
)
}
shinyApp(ui, server)
}