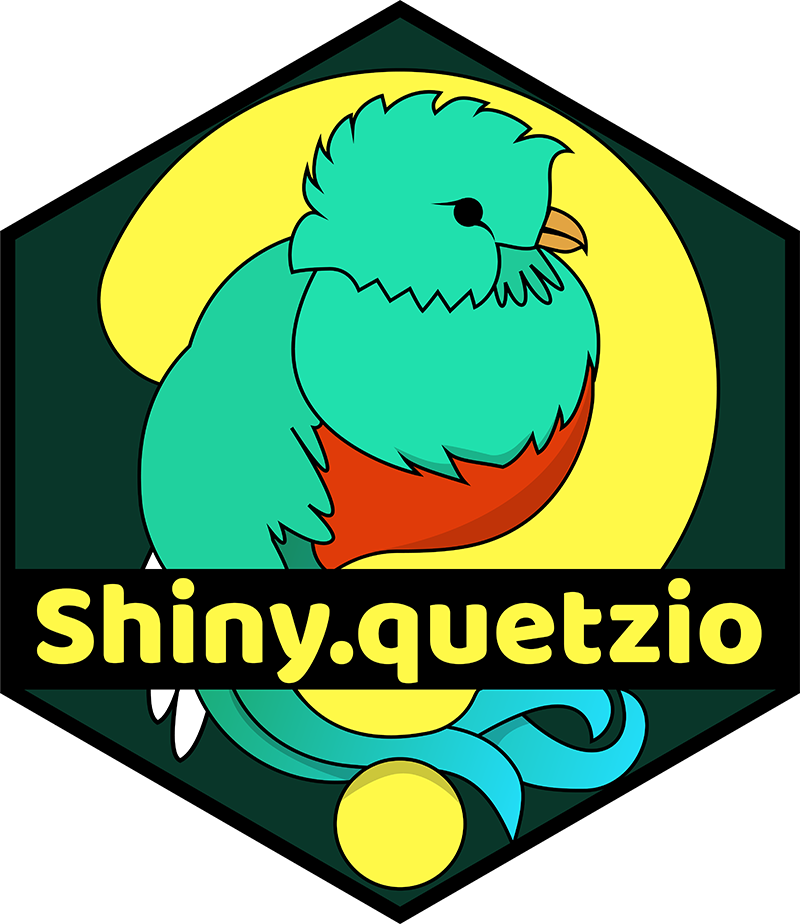
Update Quetzio selections based on external object
Quetzio_value_update.Rd
Update Quetzio selections based on external object
Usage
Quetzio_value_update(Quetzio, values, name)
# S3 method for Quetzio
Quetzio_value_update(Quetzio, values)
# S3 method for QuetzioLink
Quetzio_value_update(Quetzio, values, name)
Arguments
- Quetzio
object of class
Quetzio
orQuetzio_link
- values
list of values to update questionnaire with. List needs to be named, as the names are going to be used to identify which inputId to update
- name
string indicating in which questionnaire the questions to update are located. Used with
QuetzioLink
objects
Examples
## only run examples in interactive environment
if (interactive()) {
library(shiny)
library(shiny.quetzio)
ui <- fluidPage(
# first questionnaire to get values from
column(6,
h1("Finish first questionnaire"),
Quetzio_UI("first_questionnaire")
),
# second questionnaire to update values
column(6,
h1("Update values of second questionnaire!"),
actionButton("update_vals", "Update values"),
tags$hr(),
Quetzio_UI("second_questionnaire")
)
)
server <- function(input, output, session) {
quetzio_1st <- Quetzio_create(
source_method = "raw",
source_object = quetzio_examples$questions_lists$simple_quetzio,
module_id = "first_questionnaire"
)
quetzio_2nd <- Quetzio_create(
source_method = "raw",
source_object = quetzio_examples$questions_lists$simple_quetzio,
module_id = "second_questionnaire"
)
# update values on button press
observeEvent(input$update_vals, {
Quetzio_value_update(
Quetzio = quetzio_2nd,
# you can use answers from one questionnaire to update another, though
# the used values can be any other static named list
values = quetzio_1st$answers()
)
})
}
shinyApp(ui, server)
}
## only run examples in interactive environment
if (interactive()) {
library(shiny)
library(shiny.quetzio)
ui <- fluidPage(
# first questionnaire to get values from
column(6,
h1("Finish first questionnaire"),
Quetzio_UI("first_questionnaire")
),
# quetzio link to update values
column(6,
h1("Update values of quetzio link!"),
actionButton("update_vals", "Update values"),
tags$hr(),
QuetzioLink_UI("updating_link")
)
)
server <- function(input, output, session) {
quetzio_1st <- Quetzio_create(
source_method = "raw",
source_object = quetzio_examples$questions_lists$simple_quetzio,
module_id = "first_questionnaire"
)
quetzio_link <- QuetzioLink_create(
value_update = Quetzio_create(
source_method = "raw",
source_object = quetzio_examples$questions_lists$simple_quetzio,
module_id = "first_in_link"
),
another_one = Quetzio_create(
source_method = "raw",
source_object = quetzio_examples$questions_lists$link_quetzio_2,
module_id = "second_in_link"
),
link_id = "updating_link"
)
# update values on button press
observeEvent(input$update_vals, {
Quetzio_value_update(
Quetzio = quetzio_link,
# you need to provide quetzio name in the link to update
name = "value_update",
# you can use answers from one questionnaire to update another, though
# the used values can be any other static named list
values = quetzio_1st$answers()
)
})
}
shinyApp(ui, server)
}