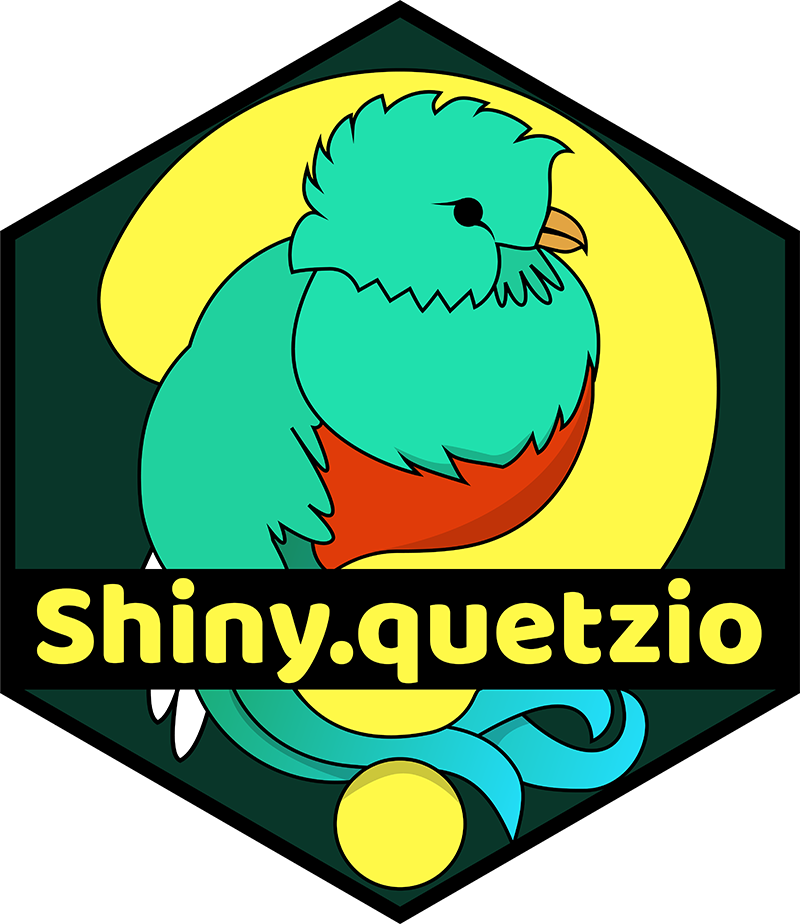
Create input for Likert scale questions based on radioButtons
likertRadioButtons.Rd
Create input for Likert scale questions based on radioButtons
Usage
likertRadioButtons(
inputId,
label,
choiceValues,
choiceNames,
selected = character(0),
placeholder = "Select value",
width = "500px"
)
Arguments
- inputId
The input slot that will be used to access the value.
- label
Label that will be shown to the user
- choiceValues
Vector with numeric values to use. Minimal length is 3. Too long scale may cause the widget to look not as intended.
- choiceNames
Character vector, indicating meaning of each choiceValue after user selects it. If you don't wish to show meaning for every value, provide character vector of length 2 with meaning of min and max
- selected
Initially selected value. Defaults to 'character(0)', which will make selection empty on init. Otherwise provide one of the values in 'choiceValues'
- placeholder
Initial text in showing meaning of selected choiceValue. Meaningful only if 'length(choiceNames) > 2' and 'selected = character(0)'. Defaults to 'Select value'
- width
Width of the input in either relative (%) or absolute (px) values.
Details
Generated input can have choiceNames character correlated to every choiceValues number - if that is the case, then the indicator showing the character associated to currently checked value will be rendered.
Alternatively, choiceNames can be a character vector of length 2, showing at all times the character associated to lowest (or the farthest to the left) and highest (or the farthest to the right) value.
In that case, the character value provided to the 'placeholder' don't matter, as it won't be generated.
Examples
## Only run in interactive session
if (interactive()) {
ui <- fluidPage(
likertRadioButtons(
inputId = "first",
label = "First input",
# you need to provide numeric value present among the choiceValues
selected = 4,
choiceValues = 1:7,
# only names for min and max (left and right)
choiceNames = c("Not much", "Many")),
verbatimTextOutput("first_output"),
likertRadioButtons(
inputId = "second",
label = "Second input",
# default (character(0)) value will make no initial selection
# selected = character(0),
choiceValues = c(-2:2),
choiceNames = c("Very bad", "Slightly bad", "Not bad or good",
"Slighty good", "Very good")),
verbatimTextOutput("second_output")
)
server <- function(input, output, session) {
output$first_output <- renderPrint(input$first)
output$second_output <- renderPrint(input$second)
}
shinyApp(ui, server)
}