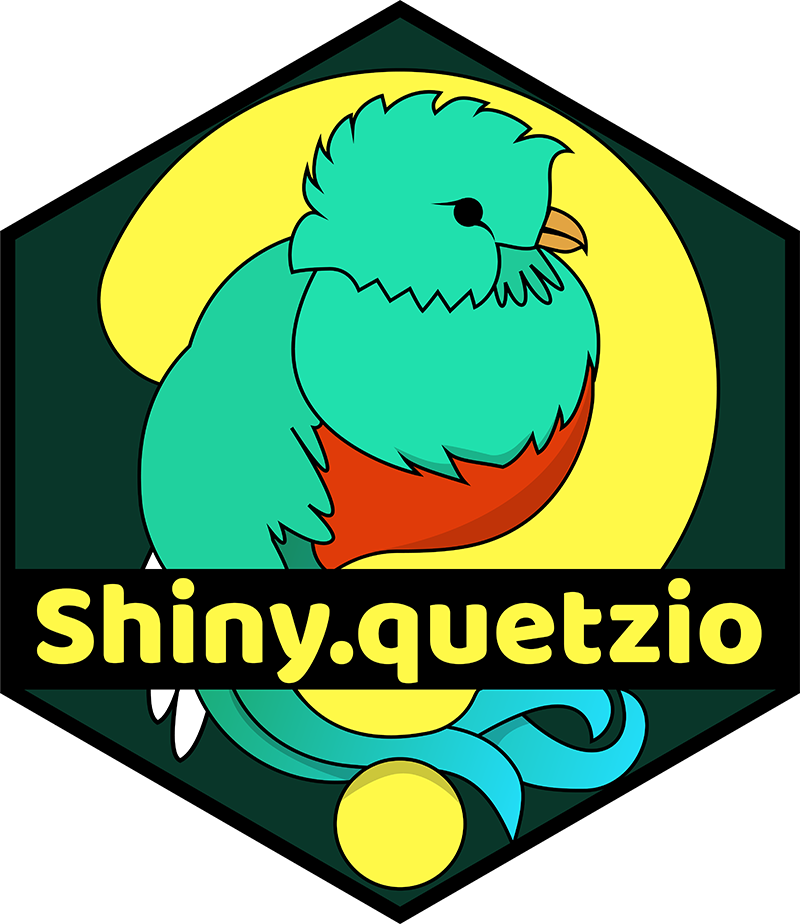
Quetzio Link Server class
QuetzioLink.Rd
Create R6-based server module to generate and hold the state of multiple linked 'Quetzio' objects
Public fields
link_id
character with id of the quetzio_link_module
completion
reactiveVal object holding the rate of linked questionnaires completion
message
reactiveVal object holding the list of linked questionnaires error messages
answers
reactiveVal object holding the list of answers to the linked questionnaires
quetzio_list
reactiveValues holding the linked 'Quetzio's
Methods
Method new()
Initialization of the 'QuetzioLink' object
Usage
QuetzioLink$new(
...,
link_id,
output_gsheet = FALSE,
output_gsheet_id = NULL,
output_gsheet_sheetname = NULL,
questionee_id = NULL
)
Arguments
...
'Quetzio' objects that are going to be connected by the link. They are all needed to be named - with the names providing the path to the answers of their questions. Moreover, they need to be in initialized state:
Quetzio$new(...)
link_id
character specifying the module ID of created object. Needs to be identical to the one specified inside every 'Quetzio' objects provided to the object.
output_gsheet
logical: do you wish to save the answers automatically to the googlesheet. If TRUE, the 'output_gsheet_id' and 'output_gsheet_sheetname' arguments need to be specified. Defaults to FALSE
output_gsheet_id
id of the output googlesheet file
output_gsheet_sheetname
name of the output spreadsheet
questionee_id
reactive object containing ID of the questionee to append to the answers list during its retrieval with
Quetzio_get_df
or sending to googlesheets. Optional.
Examples
## Only run example in interactive R sessions
if (interactive()) {
#### create shinyApp
# load libraries
library(shiny)
library(shiny.quetzio)
# create ui
ui <- fluidPage(
column(6, align = "center",
# bind the UI with correct link_id
QuetzioLink_UI("my_quetzio_link")
),
column(6,
h2("State of", tags$i("QuetzioLink")),
h3("Completion rate"),
verbatimTextOutput("quetzio_completion"),
h3("Error messages?"),
verbatimTextOutput("quetzio_message"),
h3("Answers"),
verbatimTextOutput("quetzio_answers")
)
)
server <- function(input, output, session) {
# initialize new quetzio link
linked_questionnaires <- QuetzioLink$new(
# initialize Quetzios within it (give them names!)
quetzio_first = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$link_quetzio_1,
desc_object = quetzio_examples$description_lists$link_quetzio_1,
module_id = "my_first_quetzio",
custom_css = list(
"quetzio_list" = "text-align: left; margin-left: 35%;"
)
),
quetzio_second = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$link_quetzio_2,
desc_object = quetzio_examples$description_lists$link_quetzio_2,
module_id = "my_second_quetzio",
custom_css = list(
"shiny-options-group" = "text-align: left; margin-left: 45%;"
)
),
quetzio_third = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$link_quetzio_3,
desc_object = quetzio_examples$description_lists$link_quetzio_3,
module_id = "my_thirdt_quetzio"
),
link_id = "my_quetzio_link"
)
# render objects from quetzio_link
output$quetzio_completion <-
renderPrint(linked_questionnaires$completion())
output$quetzio_message <-
renderPrint(linked_questionnaires$message())
output$quetzio_answers <-
renderPrint(linked_questionnaires$answers())
}
shinyApp(ui, server)
}
Method get_answers_df()
method to get preprocessed answers in the form of dataframe (only if the questionnaire is done)
Method update_labels()
Method to update labels on the change in reactive
Usage
QuetzioLink$update_labels(
quetzio_name,
trigger,
source_method,
source_yaml = NULL,
source_gsheet_id = NULL,
source_gsheet_sheetname = NULL,
source_object = NULL
)
Arguments
quetzio_name
string indicating in which questionnaire the questions to update are located
trigger
reactive which will trigger the update. It needs to take values linked to the changes in the source
source_method
character string specifying in what form the source config file will be provided. Can be either 'gsheet', 'yaml' or 'raw'. Necessity of other arguments is dependent on this choice. For more info see 'details'
source_yaml
path to the source yaml file
source_gsheet_id
id of the source googlesheet file
source_gsheet_sheetname
name of the source spreadsheet
source_object
object of class
list
(similiar in structure to 'yaml' source) ordata.frame
(similiar in structure to 'googlesheet' source) to be the source of questions. You can create a sample data.frame withcreate_survey_source()
. Needed whensource_method == 'raw'
Examples
## only run examples in interactive environment
if (interactive()) {
library(shiny)
library(shiny.quetzio)
ui <- fluidPage(
# some input to trigger label update
selectizeInput("gender", "What is your gender?",
choices = c("Male" = "M",
"Female" = "F",
"I identify as neither of above" = "O",
"Prefer not to say" = "NI"),
selected = "NI"),
tags$hr(),
# quetzio to update labels
QuetzioLink_UI("labels_link")
)
server <- function(input, output, session) {
quetzio_link <- QuetzioLink$new(
gender = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$gender_update,
module_id = "updating_labels"
),
quetzio_2nd = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$simple_quetzio,
module_id = "second_in_link"
),
link_id = "labels_link")
# trigger need to be reactive
gender_react <- reactive(input$gender)
# update labels method call
quetzio_link$update_labels(
# you need to provide the name of the Quetzio in link
# where you need to update labels
quetzio_name = "gender",
# the trigger needs to be reactive, but without the parentheses
trigger = gender_react,
source_method = "raw",
source_object = quetzio_examples$label_update$gender_update
)
}
shinyApp(ui, server)
}
Method update_values()
Method to update selected values on the change in reactive
Arguments
quetzio_name
string indicating in which questionnaire the item values to update are located
values
list of values to update questionnaire with. List needs to be named, as the names are going to be used to identify which inputId to update
Examples
## only run examples in interactive environment
if (interactive()) {
library(shiny)
library(shiny.quetzio)
ui <- fluidPage(
# first questionnaire to get values from
column(6,
h1("Finish first questionnaire"),
Quetzio_UI("first_questionnaire")
),
# quetzio link to update values
column(6,
h1("Update values of quetzio link!"),
actionButton("update_vals", "Update values"),
tags$hr(),
QuetzioLink_UI("updating_link")
)
)
server <- function(input, output, session) {
quetzio_1st <- Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$simple_quetzio,
module_id = "first_questionnaire"
)
quetzio_link <- QuetzioLink$new(
value_update = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$simple_quetzio,
module_id = "first_in_link"
),
another_one = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$link_quetzio_2,
module_id = "second_in_link"
),
link_id = "updating_link"
)
# update values on button press
observeEvent(input$update_vals, {
quetzio_link$update_values(
# you need to provide quetzio name in the link to update
quetzio_name = "value_update",
# you can use answers from one questionnaire to update another, though
# the used values can be any other static named list
values = quetzio_1st$answers()
)
})
}
shinyApp(ui, server)
}
Examples
## ------------------------------------------------
## Method `QuetzioLink$new`
## ------------------------------------------------
## Only run example in interactive R sessions
if (interactive()) {
#### create shinyApp
# load libraries
library(shiny)
library(shiny.quetzio)
# create ui
ui <- fluidPage(
column(6, align = "center",
# bind the UI with correct link_id
QuetzioLink_UI("my_quetzio_link")
),
column(6,
h2("State of", tags$i("QuetzioLink")),
h3("Completion rate"),
verbatimTextOutput("quetzio_completion"),
h3("Error messages?"),
verbatimTextOutput("quetzio_message"),
h3("Answers"),
verbatimTextOutput("quetzio_answers")
)
)
server <- function(input, output, session) {
# initialize new quetzio link
linked_questionnaires <- QuetzioLink$new(
# initialize Quetzios within it (give them names!)
quetzio_first = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$link_quetzio_1,
desc_object = quetzio_examples$description_lists$link_quetzio_1,
module_id = "my_first_quetzio",
custom_css = list(
"quetzio_list" = "text-align: left; margin-left: 35%;"
)
),
quetzio_second = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$link_quetzio_2,
desc_object = quetzio_examples$description_lists$link_quetzio_2,
module_id = "my_second_quetzio",
custom_css = list(
"shiny-options-group" = "text-align: left; margin-left: 45%;"
)
),
quetzio_third = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$link_quetzio_3,
desc_object = quetzio_examples$description_lists$link_quetzio_3,
module_id = "my_thirdt_quetzio"
),
link_id = "my_quetzio_link"
)
# render objects from quetzio_link
output$quetzio_completion <-
renderPrint(linked_questionnaires$completion())
output$quetzio_message <-
renderPrint(linked_questionnaires$message())
output$quetzio_answers <-
renderPrint(linked_questionnaires$answers())
}
shinyApp(ui, server)
}
## ------------------------------------------------
## Method `QuetzioLink$update_labels`
## ------------------------------------------------
## only run examples in interactive environment
if (interactive()) {
library(shiny)
library(shiny.quetzio)
ui <- fluidPage(
# some input to trigger label update
selectizeInput("gender", "What is your gender?",
choices = c("Male" = "M",
"Female" = "F",
"I identify as neither of above" = "O",
"Prefer not to say" = "NI"),
selected = "NI"),
tags$hr(),
# quetzio to update labels
QuetzioLink_UI("labels_link")
)
server <- function(input, output, session) {
quetzio_link <- QuetzioLink$new(
gender = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$gender_update,
module_id = "updating_labels"
),
quetzio_2nd = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$simple_quetzio,
module_id = "second_in_link"
),
link_id = "labels_link")
# trigger need to be reactive
gender_react <- reactive(input$gender)
# update labels method call
quetzio_link$update_labels(
# you need to provide the name of the Quetzio in link
# where you need to update labels
quetzio_name = "gender",
# the trigger needs to be reactive, but without the parentheses
trigger = gender_react,
source_method = "raw",
source_object = quetzio_examples$label_update$gender_update
)
}
shinyApp(ui, server)
}
## ------------------------------------------------
## Method `QuetzioLink$update_values`
## ------------------------------------------------
## only run examples in interactive environment
if (interactive()) {
library(shiny)
library(shiny.quetzio)
ui <- fluidPage(
# first questionnaire to get values from
column(6,
h1("Finish first questionnaire"),
Quetzio_UI("first_questionnaire")
),
# quetzio link to update values
column(6,
h1("Update values of quetzio link!"),
actionButton("update_vals", "Update values"),
tags$hr(),
QuetzioLink_UI("updating_link")
)
)
server <- function(input, output, session) {
quetzio_1st <- Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$simple_quetzio,
module_id = "first_questionnaire"
)
quetzio_link <- QuetzioLink$new(
value_update = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$simple_quetzio,
module_id = "first_in_link"
),
another_one = Quetzio$new(
source_method = "raw",
source_object = quetzio_examples$questions_lists$link_quetzio_2,
module_id = "second_in_link"
),
link_id = "updating_link"
)
# update values on button press
observeEvent(input$update_vals, {
quetzio_link$update_values(
# you need to provide quetzio name in the link to update
quetzio_name = "value_update",
# you can use answers from one questionnaire to update another, though
# the used values can be any other static named list
values = quetzio_1st$answers()
)
})
}
shinyApp(ui, server)
}